Edge modes
Clamp to edge
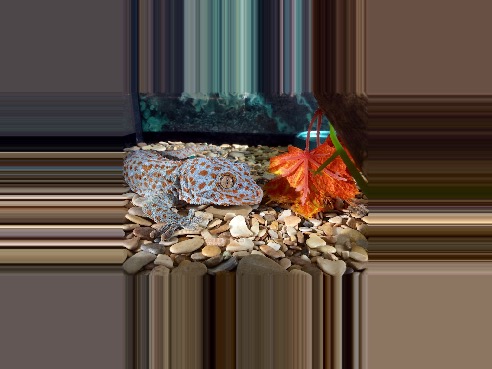
The wrap mode of image sampling when a pixel with out of range coordinates is got from the corresponding border.
x = clamp(x, 0, w - 1); y = clamp(y, 0, h - 1);
Clamp to zero
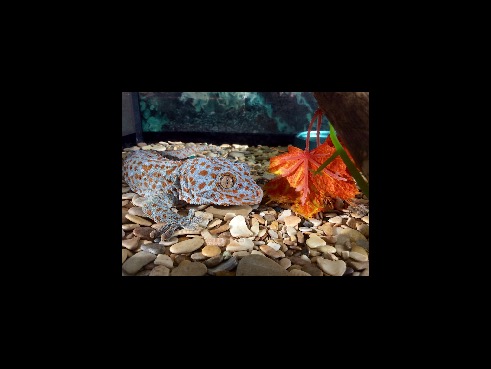
The wrap mode of image sampling when a pixel with out of range coordinates is got as zero.
if (x < 0 || x >= w || y < 0 || y >= h) return 0;
Repeat
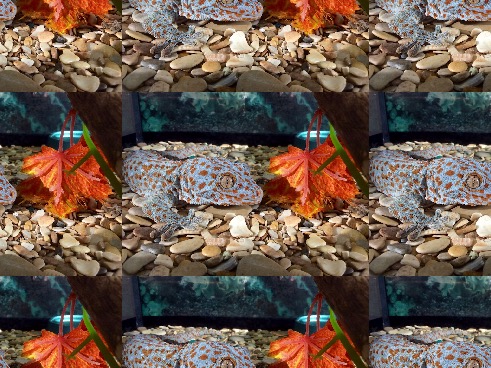
The wrap mode of image sampling when a pixel with out of range coordinates is got from the corresponding coordinates of next tile of the same image.
x = (x + w) % w; y = (y + h) % h;
Mirrored Repeat
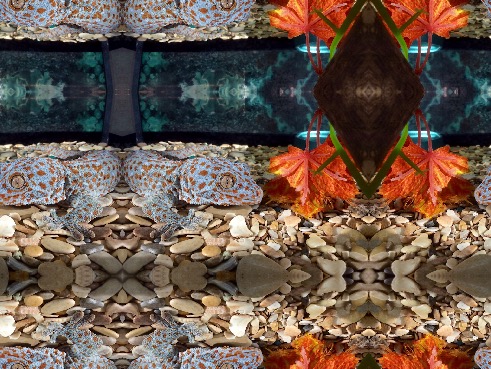
The wrap mode of image sampling when a pixel with out of range coordinates is got from the corresponding coordinates of next tile of the same image, but every odd tile is mirrored. Math explanation in Desmos.
float s(x, l) { if (x == 0) return 1; if (x % l == 0) return 0; return int(ceil(abs(x) / float(l))) % 2; } xw = s(x, w); yh = s(y, h); x = (abs(x) % w) * (xw * 2 - 1) + (w - 1) * (1 - xw); y = (abs(y) % h) * (yh * 2 - 1) + (h - 1) * (1 - yh);
Filtering
The filtering mode determines how pixels of source image will be combined in result of sampling. There are several modes, but here only two will be considered: nearest and linear.
In the nearest mode, there are returned the value of the image element that is nearest (in Manhattan distance) to the center of the pixel.
x = round(x); y = round(y); return _get_pixel(image, x, y);
In the linear mode, there are returned the weighted average of the four image elements that are closest to the center of the pixel being.
l = floor(x); r = ceil(x); t = floor(y); b = ceil(y); h_coef = x - l; v_coef = y - t; tl = _get_pixel(image, l, t); tr = _get_pixel(image, r, t); bl = _get_pixel(image, l, b); br = _get_pixel(image, r, b); return mix(mix(tl, tr, h_coef), mix(bl, br, h_coef), v_coef);
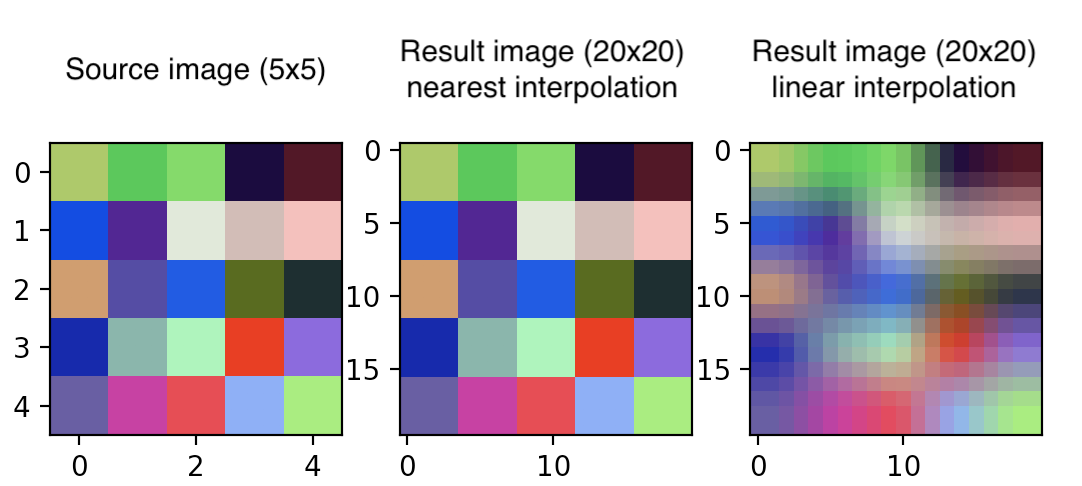
Moreover, there is some nuance with reading a pixel from another scale. The following illustration and math equation can help understand the problem.
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 0 | 1 | 2 | 3 0 | 1 NewCoord = (Coord + 0.5) * Scale - 0.5